一、 功能
Android端或者Android終端的遠程截圖至本地電腦中
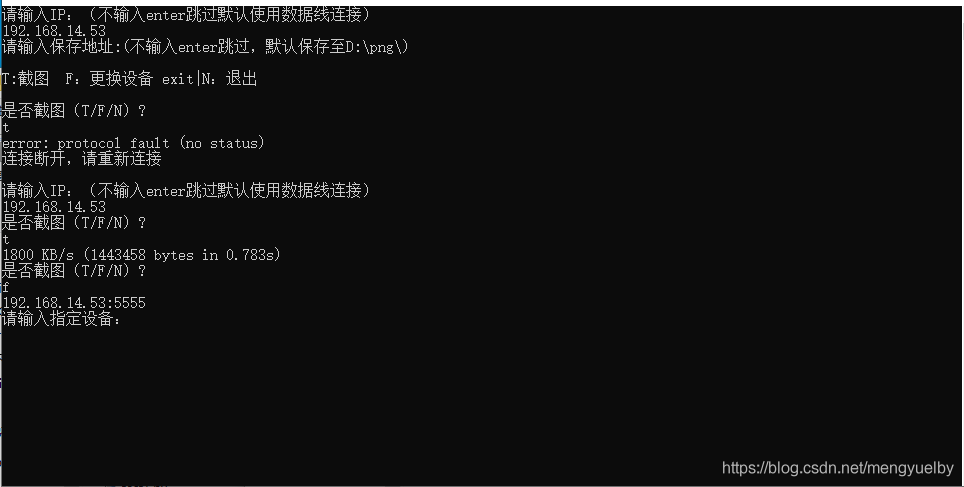
二、使用說明
1.adb截圖工具可用于Android手機及Android終端
2.使用數據線連接前提:電腦與Android終端/手機已連接
Android終端/手機已打開開發者模式
3.生成的圖片格式為png
三、實現
1.初始源碼
import time
import os,sys
#截圖
def screencap_cmd(filePath,devices=None):
if filePath==None:
filePath='D:/png/'
if not os.path.exists(filePath):
os.makedirs(filePath)
filename=time.strftime("%Y%m%d%H%M%S",time.localtime())+".png"
if devices==None:
cmd_screencap='adb shell screencap -p sdcard/'+filename
cmd_pull='adb pull sdcard/'+filename+' '+filePath+filename
cmd_delete='adb shell rm sdcard/'+filename
else:
cmd_screencap='adb -s '+devices+' shell screencap -p sdcard/'+filename
cmd_pull='adb -s '+devices+' pull sdcard/'+filename+' '+filePath+filename
cmd_delete='adb -s '+devices+' shell rm sdcard/'+filename
os.system(cmd_screencap)
os.system(cmd_pull)
os.system(cmd_delete)
#保存地址判斷及設備信息分類調用
def screencap_info(devices=None,filePath=None):
if filePath==None:
filePath='D:/png/'
if not os.path.exists(filePath):
os.makedirs(filePath)
if devices==None:
screencap_cmd(filePath)
adb_device_none(filePath)
else:
screencap_cmd(filePath,devices)
adb_info(devices,filePath)
#更換設備
def change_devices():
r=os.popen("adb devices")
lines=r.readlines()
lines=lines[1:-1]
n=len(lines)
for i in lines:
print(i.split("\t")[0])
devices=input("請輸入指定設備:\n")
for i in lines:
if devices in i:
return devices
print("未找到改設備請重新輸入!")
change_devices()
#截圖命令判斷,電腦連接多個設備或使用IP截圖時
def adb_info(lines,filePath=None):
s=input("是否截圖(T/F/N)?\n")
if s=='t' or s=='T':
if filePath==None:
screencap_info(lines)
else:
screencap_info(lines,filePath)
elif s=='N' or s=='n' or s=='exit':
sys.exit()
elif s=='F' or s=='f':
devices=change_devices()
if filePath==None:
adb_info(devices)
else:
adb_info(devices,filePath)
#截圖命令判斷,電腦連接僅連接一個設備時
def adb_device_none(filePath=None):
s=input("是否截圖(T/F/N)?\n")
if s=='t' or s=='T':
if filePath==None:
screencap_info()
else:
screencap_info(None,filePath)
elif s=='N' or s=='n' or s=='exit':
sys.exit()
elif s=='F' or s=='f':
devices=change_devices()
if filePath==None:
adb_info(devices)
else:
adb_info(devices,filePath)
#使用設備判斷
def adb_devices(n,lines,filePath=None):
if n==0:
print("請檢查是否已接連或啟動調試模式或安裝adb環境")
s=input("再次啟動(T/F)?\n")
if s=='t' or s=='T':
r=os.popen("adb devices")
lines=r.readlines()
lines=lines[1:-1]
n=len(lines)
adb_devices(n,r.readlines())
else:
sys.exit()
elif ":5555" in lines:
print("T:截圖 F:更換設備 exit|N:退出 ")
if filePath==None:
adb_info(lines)
else:
adb_info(lines,filePath)
elif n==1:
print("T:截圖 F:更換設備 exit|N:退出")
if filePath==None:
adb_device_none()
else:
adb_device_none(filePath)
elif n>1:
for i in lines:
print(i.split("\t")[0])
devices=input("請輸入指定設備:\n")
for i in lines:
if devices in i:
print("T:截圖 F:更換設備 exit|N:退出")
if filePath==None:
adb_info(devices)
else:
adb_info(devices,filePath)
print("未找到改設備請重新輸入!")
if filePath==None:
adb_devices(n,lines)
else:
adb_devices(n,lines,filePath)
#輸入IP
def ip_info():
ipPath=input("請輸入IP:(不輸入enter跳過默認使用數據線連接)\n")
if len(ipPath)>0:
try:
cnd='adb connect '+ipPath
os.system(cnd)
return ipPath
except:
print("ADB調試模式為USB,需要切換到無線調試模式\n")
print("切換方法:\n第一步:Android設備開啟USB調試,并且通過USB線連接到電腦\n第二步:在終端執行以下命令”adb tcpip 5555“\n第三步:在終端執行以下命令”adb connect ip“。此時拔出USB線,應該就可以adb通過wifi調試設備\n")
return ip_info()
if __name__ == '__main__':
ipPath=ip_info()
filePath=input("請輸入保存地址:(不輸入enter跳過,默認保存至D:\png\)\n")
#查詢當前連接的設備
r=os.popen("adb devices")
lines=r.readlines()
lines=lines[1:-1]
n=len(lines)
ip_add=0
if ipPath!=None:
for i in lines:
if ipPath in i:
ip_add=i.split("\t")[0]
if len(filePath)>0:
if ":\\" in filePath:
if ip_add!=0:
adb_devices(n,ip_add,filePath)
else:
adb_devices(n,lines,filePath)
else:
print("地址輸入非法,使用默認地址\n")
if ip_add!=0:
adb_devices(n,ip_add)
else:
adb_devices(n,lines)
else:
if ip_add!=0:
adb_devices(n,ip_add)
else:
adb_devices(n,lines)
2.優化:增加ip連接斷開重連處理
在輸入ip函數ip_info()增加ip連接判斷,將os.system替換成os.popen函數執行cmd命令:
r=os.popen(cnd)
#讀取執行結果
lines=r.readlines()[0]
if 'unable' in lines:
print("連接失敗\n")
return ip_info()
else:
return ipPath
- os.system函數無法獲取執行結果,只能獲取執行成功或失敗的結果,成功返回0,失敗返回1,且會執行的結果打印在終端上,相當于調起dos執行命令
- os.popen函數可獲取執行的結果內容,但獲取的結果要進行readlines讀取,執行的結果不會打印在終端上
在輸入ip函數ip_info()增加ip連接判斷,將os.system替換成os.popen函數執行cmd命令:
a=os.system(cmd_screencap)
if a==0:
os.system(cmd_pull)
os.system(cmd_delete)
else:
if ":5555" in devices:
print("連接斷開,請重新連接\n")
ipPath=ip_info()
if ipPath==None:
ipPath=ip_info()
else:
device=ipPath+":5555"
adb_info(device,filePath)
else:
print("設備連接斷開,請更換設備\n")
device=change_devices()
adb_info(device,filePath)
3.最終源碼
import time
import os,sys
#輸入IP
def ip_info():
ipPath=input("請輸入IP:(不輸入enter跳過默認使用數據線連接)\n")
if len(ipPath)>0:
try:
cnd='adb connect '+ipPath
r=os.popen(cnd)
lines=r.readlines()[0]
if 'unable' in lines:
print("連接失敗\n")
return ip_info()
else:
return ipPath
except:
print("ADB調試模式為USB,需要切換到無線調試模式\n")
print("切換方法:\n第一步:Android設備開啟USB調試,并且通過USB線連接到電腦\n第二步:在終端執行以下命令”adb tcpip 5555“\n第三步:在終端執行以下命令”adb connect ip“。此時拔出USB線,應該就可以adb通過wifi調試設備\n")
return ip_info()
#保存地址判斷及設備信息分類調用
def screencap_info(devices=None,filePath=None):
if filePath==None:
filePath='D:/png/'
if not os.path.exists(filePath):
os.makedirs(filePath)
if devices==None:
screencap_cmd(filePath)
adb_device_none(filePath)
else:
screencap_cmd(filePath,devices)
adb_info(devices,filePath)
#更換設備
def change_devices():
r=os.popen("adb devices")
lines=r.readlines()
lines=lines[1:-1]
n=len(lines)
for i in lines:
print(i.split("\t")[0])
devices=input("請輸入指定設備:\n")
for i in lines:
if devices in i:
return devices
print("未找到改設備請重新輸入!\n")
change_devices()
#截圖命令判斷,電腦連接多個設備或使用IP截圖時
def adb_info(lines,filePath=None):
s=input("是否截圖(T/F/N)?\n")
if s=='t' or s=='T':
if filePath==None:
screencap_info(lines)
else:
screencap_info(lines,filePath)
elif s=='N' or s=='n' or s=='exit':
sys.exit()
elif s=='F' or s=='f':
devices=change_devices()
if filePath==None:
adb_info(devices)
else:
adb_info(devices,filePath)
#截圖命令判斷,電腦連接僅連接一個設備時
def adb_device_none(filePath=None):
s=input("是否截圖(T/F/N)?\n")
if s=='t' or s=='T':
if filePath==None:
screencap_info()
else:
screencap_info(None,filePath)
elif s=='N' or s=='n' or s=='exit':
sys.exit()
elif s=='F' or s=='f':
devices=change_devices()
if filePath==None:
adb_info(devices)
else:
adb_info(devices,filePath)
#使用設備判斷
def adb_devices(n,lines,filePath=None):
if n==0:
print("請檢查是否已接連或啟動調試模式或安裝adb環境\n")
s=input("再次啟動(T/F)?\n")
if s=='t' or s=='T':
r=os.popen("adb devices")
lines=r.readlines()
lines=lines[1:-1]
n=len(lines)
adb_devices(n,r.readlines())
else:
sys.exit()
elif ":5555" in lines:
print("T:截圖 F:更換設備 exit|N:退出\n")
if filePath==None:
adb_info(lines)
else:
adb_info(lines,filePath)
elif n==1:
print("T:截圖 F:更換設備 exit|N:退出\n")
if filePath==None:
adb_device_none()
else:
adb_device_none(filePath)
elif n>1:
for i in lines:
print(i.split("\t")[0])
devices=input("請輸入指定設備:\n")
for i in lines:
if devices in i:
print("T:截圖 F:更換設備 exit|N:退出")
if filePath==None:
adb_info(devices)
else:
adb_info(devices,filePath)
print("未找到改設備請重新輸入!")
if filePath==None:
adb_devices(n,lines)
else:
adb_devices(n,lines,filePath)
#截圖
def screencap_cmd(filePath,devices=None):
if filePath==None:
filePath='D:/png/'
if not os.path.exists(filePath):
os.makedirs(filePath)
filename=time.strftime("%Y%m%d%H%M%S",time.localtime())+".png"
if devices==None:
cmd_screencap='adb shell screencap -p sdcard/'+filename
cmd_pull='adb pull sdcard/'+filename+' '+filePath+filename
cmd_delete='adb shell rm sdcard/'+filename
else:
cmd_screencap='adb -s '+devices+' shell screencap -p sdcard/'+filename
cmd_pull='adb -s '+devices+' pull sdcard/'+filename+' '+filePath+filename
cmd_delete='adb -s '+devices+' shell rm sdcard/'+filename
a=os.system(cmd_screencap)
if a==0:
os.system(cmd_pull)
os.system(cmd_delete)
else:
if ":5555" in devices:
print("連接斷開,請重新連接\n")
ipPath=ip_info()
if ipPath==None:
ipPath=ip_info()
else:
device=ipPath+":5555"
adb_info(device,filePath)
else:
print("設備連接斷開,請更換設備\n")
device=change_devices()
adb_info(device,filePath)
if __name__ == '__main__':
ipPath=ip_info()
filePath=input("請輸入保存地址:(不輸入enter跳過,默認保存至D:\png\)\n")
#查詢當前連接的設備
r=os.popen("adb devices")
lines=r.readlines()
lines=lines[1:-1]
n=len(lines)
ip_add=0
if ipPath!=None:
for i in lines:
if ipPath in i:
ip_add=i.split("\t")[0]
if len(filePath)>0:
if ":\\" in filePath:
if ip_add!=0:
adb_devices(n,ip_add,filePath)
else:
adb_devices(n,lines,filePath)
else:
print("地址輸入非法,使用默認地址\n")
if ip_add!=0:
adb_devices(n,ip_add)
else:
adb_devices(n,lines)
else:
if ip_add!=0:
adb_devices(n,ip_add)
else:
adb_devices(n,lines)
到此這篇關于python編寫adb截圖工具的文章就介紹到這了,更多相關python adb截圖工具內容請搜索腳本之家以前的文章或繼續瀏覽下面的相關文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- Python腳本利用adb進行手機控制的方法
- python3 adb 獲取設備序列號的實現
- python+adb命令實現自動刷視頻腳本案例
- 通過python調用adb命令對App進行性能測試方式
- 通過Python實現控制手機詳解